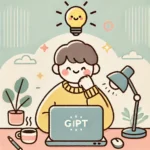
「LaravelでgbizとAPI連携をやってみたいけど、どこから始めればいいの?」「公式ドキュメントだけでは分かりにくい…」そう思う方もいるかもしれません。
Laravelを使えば、gbizのAPIとスムーズに連携できますが、手順を理解しておかないとつまずくポイントもあります。
この記事では、Laravelとgbiz APIを連携する具体的な手順や設定方法、開発のコツを分かりやすく解説します。
本記事のテーマ
LaravelでgBizINFOとAPI連携をやってみた!
本記事を読むと分かること
- gbizとのAPI連携方法
未経験からエンジニアを目指す方は下記プログラミングスクールがおすすめ!

1. gBizINFOとは
概要
法人として登録されている約400万社の企業情報を検索、閲覧できるサイトになります。
詳細は下記サイトから確認をお願いします。
gBizINFOについて | gBizINFO
法人番号を持っている約400万社を対象とし、法人名、本社所在地に加えて、契約情報、表彰情報等の府省庁が保有・公開している情報を一括検索、閲覧できます。
API利用方法
下記サイトからAPIの仕様や利用申請が出来ますので、申請をお願いします。
API利用方法 | gBizINFO
法人番号を持っている約400万社を対象とし、法人名、本社所在地に加えて、契約情報、表彰情報等の府省庁が保有・公開している情報を一括検索、閲覧できます。
申請が通るとAPIトークンが取得できます!

2. LaravelでgBizINFOのAPIを叩く
Laravelで取得したAPI連携を行っていきます!
実際の画面
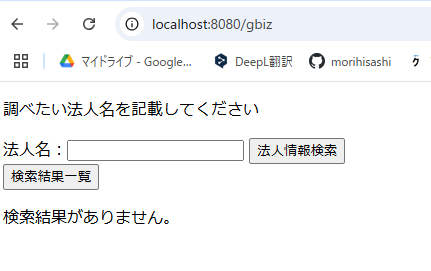
法人情報検索を行うと
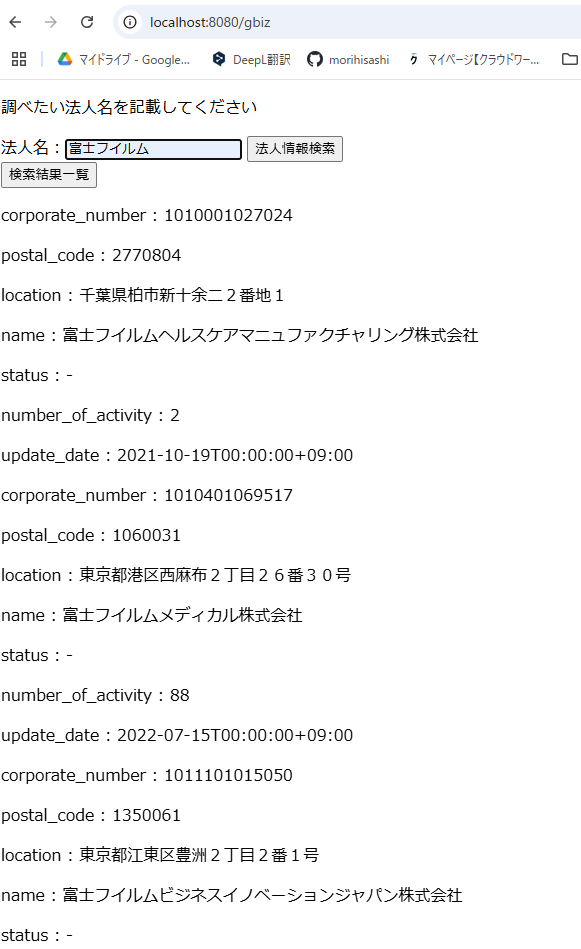
下に検索に引っ掛かった企業を返すようにしていきます。
controller作成
controllerではviewのactionを受けとってmodelにrequest内容を渡しております
GbizController.php
<?php
namespace App\Http\Controllers;
use Illuminate\Foundation\Auth\Access\AuthorizesRequests;
use Illuminate\Foundation\Bus\DispatchesJobs;
use Illuminate\Foundation\Validation\ValidatesRequests;
use Illuminate\Routing\Controller as BaseController;
use Illuminate\Http\RedirectResponse;
use Illuminate\Http\Request;
use Illuminate\View\View;
use App\Models\Gbiz;
use Illuminate\Support\Facades\Log;
use function PHPUnit\Framework\isNull;
class GbizController extends Controller
{
use AuthorizesRequests, DispatchesJobs, ValidatesRequests;
public function index(): View
{
$data = '';
return view('gbiz.index')->with('data', $data);
}
public function redirect(Request $request): View
{
// リクエストデータの処理
$data = '';
if(!empty($request->input('name'))){
$name = $request->input('name');
// modelを呼び出す
$gbiz = new Gbiz();
$res = $gbiz->getApi($name);
// 不要な部分を削除し、JSON部分のみを抽出
$jsonString = substr($res, strpos($res, '{'));
Log::info($jsonString);
// gbiz_searchへ取得結果を保存する
$result = $gbiz->insertApiData($name, $jsonString);
if($result){
Log::info('gbiz_searchへのinsertが完了しました。');
}
// JSON文字列をPHPの配列に変換
$data = json_decode($jsonString, true);
return view('gbiz.index')->with('data', $data);
}
// アサイン
return view('gbiz.index')->with('data', $data);
}
// 検索結果の一覧表示
public function list(Request $request): View
{
// modelを呼び出す
$gbiz = new Gbiz();
$list = $gbiz->getData();
if(count($list) === 0){
$list = '';
}
// アサイン
return view('gbiz.list')->with('list', $list);
}
// 検索結果の詳細表示
public function detail(Request $request): View
{
$detail = '';
$detail = $request->input('result');
// JSON文字列をPHPの配列に変換
$detail = json_decode($detail, true);
// アサイン
return view('gbiz.detail')->with('detail', $detail["hojin-infos"]);
}
}
modelの作成
modelでは実際にAPIを叩いて、企業情報を取得しcontrollerに返してます
Gbiz.php
<?php
namespace App\Models;
require '../vendor/autoload.php';
use Dotenv\Dotenv;
use Dotenv\Exception\InvalidPathException;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Database\Eloquent\Model;
use Illuminate\Support\Facades\DB;
use Illuminate\Support\Facades\Log;
class Gbiz extends Model
{
use HasFactory;
// 検索した企業名でAPIを叩き、情報を取得する
public function getApi($name){
try {
$dotenv = Dotenv::createImmutable(__DIR__ . '/../..');
$dotenv->load();
} catch (InvalidPathException $e) {
echo 'Error loading .env file: ', $e->getMessage();
exit;
}
if(isset($name)){
$encodedString = urlencode($name);
$url = env('API_URL') . '?name=' . $encodedString . '&page=' . env('PAGE') . '&limit=' . env('LIMIT');
}
$headers = [
"Content-Type: application/json",
"X-hojinInfo-api-token: " . env('API_TOKEN'),
];
$ch = curl_init();
// オプション
curl_setopt($ch, CURLOPT_URL, $url);
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
// URLの情報を取得する
$res = curl_exec($ch);
curl_close($ch);
return $res;
}
// 取得した結果をデータベースに保存する
public function insertApiData($name, $jsonString){
if($name != ''){
try {
DB::table('gbiz_search')->insert([
'companyname' => $name,
'res' => true,
'result' => $jsonString,
'datecrt' => NOW(),
]);
Log::info('gbiz_searchにinsertを開始しました。');
return true;
} catch (InvalidPathException $e) {
echo 'Error insert gbiz_search : ', $e->getMessage();
exit;
}
}else{
return false;
}
}
// データベース保存した結果を取得する
public function getData(){
try {
$list = DB::table('gbiz_search')->get();
Log::info('gbiz_searchから情報を取得しました。');
return $list;
} catch (InvalidPathException $e) {
echo 'Error select gbiz_search : ', $e->getMessage();
exit;
}
}
}
viewの作成
viewでは最初にお見せしたような画面を表示しております。
index.blade.php
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>gbiz</title>
</head>
<script>
</script>
<body>
<form action="{{route('gbiz.redirect')}}" method="POST">
@csrf
<p>調べたい法人名を記載してください</p>
<label>法人名:<input type="text" name="name"></label>
<button type='submit' id='gbiz'>法人情報検索</button>
</form>
<form action="{{route('gbiz.list')}}" method="POST">
@csrf
<button type='submit' id='gbizlist'>検索結果一覧</button>
</form>
@if($data !== '' && isset($data["hojin-infos"]))
@foreach($data["hojin-infos"] as $value)
@foreach($value as $key => $val)
<p>{{$key}} : {{$val}}<br></p>
@endforeach
@endforeach
@else
<p>検索結果がありません。</p>
@endif
</body>
</html>
redirect.blade.php
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>gbiz</title>
</head>
<script>
// ボタンのクリックイベントを設定
function gbiz() {
// ランダムな整数を生成
let randomNumber = getRandomInt(1, 100);
console.log(randomNumber);
}
function getRandomInt(minval, maxval){
let min = Math.ceil(minval);
let max = Math.floor(maxval);
return Math.floor(Math.random() * (max - min + 1)) + min;
}
</script>
<body>
<form action="{{ route('gbiz.redirect') }}" method="POST">
@csrf
<label>法人番号:<input type="text" name="corporate_number"></label>
<label>法人名:<input type="text" name="name"></label>
<button type='submit' id='gbiz' onclick="gbiz()">法人情報検索</button>
</form>
</body>
</html>
redirect.blade.phpは無くても動くかも
routeの作成
表示するrouteの設定をします。
web.php
<?php
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\GbizController;
/*
|--------------------------------------------------------------------------
| Web Routes
|--------------------------------------------------------------------------
|
| Here is where you can register web routes for your application. These
| routes are loaded by the RouteServiceProvider within a group which
| contains the "web" middleware group. Now create something great!
|
*/
Route::get('/', function () {
return view('welcome');
});
Route::get('/gbiz', [GbizController::class, 'index'])->name('gbiz.index');
Route::post('/gbiz', [GbizController::class, 'redirect'])->name('gbiz.redirect');
Route::post('/gbiz/list', [GbizController::class, 'list'])->name('gbiz.list');
Route::post('/gbiz/detail', [GbizController::class, 'detail'])->name('gbiz.detail');
さいごに
実際に書いてみないと分からないこともあると思いますので、とりあえず書いていくことが大事だと思います!私のコードも分かりにくい箇所はあるかと思いますがご了承ください。
ここまで読んで下さり誠にありがとうございます!
コメント